Webサイトやブログにフリーな地図を表示
サイトやブログに地図を配置出来るJavaScriptで出来たLeafletを使って見ます。
基本的な地図の表示
leaflet1.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Leflet1</title>
<link rel="stylesheet" href="https://unpkg.com/leaflet@1.7.1/dist/leaflet.css"
integrity="sha512-xodZBNTC5n17Xt2atTPuE1HxjVMSvLVW9ocqUKLsCC5CXdbqCmblAshOMAS6/keqq/sMZMZ19scR4PsZChSR7A=="
/>
<script src="https://unpkg.com/leaflet@1.7.1/dist/leaflet.js"
integrity="sha512-XQoYMqMTK8LvdxXYG3nZ448hOEQiglfqkJs1NOQV44cWnUrBc8PkAOcXy20w0vlaXaVUearIOBhiXZ5V3ynxwA=="
></script>
<script>
function init() {
var map = L.map('map1');
var mpoint = [35.6812362,139.7671248];
map.setView(mpoint, 16);
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
attribution: '© <a href="https://www.openstreetmap.org/copyright" target='_blank'>OpenStreetMap</a> contributors'
}).addTo(map);
var marker = L.marker(mpoint).addTo(map);
}
</script>
</head>
<body onload="init()">
<div id="map1" style="height:600px; width:95%; text-align: center;"></div>
</body>
</html>
leafletの読み込み
・leaflet.cssを読み込んでからleaflet.jsを読み込んだ方が良いみたいです。
・integrity=”~~~”は、無くても機能します。本家以外からダウンロードした際は、一度試した方が良いかもしれません。
・leaflet.cssを読み込んでからleaflet.jsを読み込んだ方が良いみたいです。
・integrity=”~~~”は、無くても機能します。本家以外からダウンロードした際は、一度試した方が良いかもしれません。
<link rel="stylesheet" href="https://unpkg.com/leaflet@1.7.1/dist/leaflet.css"
integrity="sha512-xodZBNTC5n17Xt2atTPuE1HxjVMSvLVW9ocqUKLsCC5CXdbqCmblAshOMAS6/keqq/sMZMZ19scR4PsZChSR7A=="
/>
<script src="https://unpkg.com/leaflet@1.7.1/dist/leaflet.js"
integrity="sha512-XQoYMqMTK8LvdxXYG3nZ448hOEQiglfqkJs1NOQV44cWnUrBc8PkAOcXy20w0vlaXaVUearIOBhiXZ5V3ynxwA=="
></script>
HTMLのdivを地図にセット
・HTMLの「div id=”map1“」で付けたidをLeafletのmapにセットする。
・HTMLの「div id=”map1“」で付けたidをLeafletのmapにセットする。
var map = L.map('map1');
緯度、経度の順と倍率指定
・経緯度の指定方法は左手系経緯度で「緯度,経度」の順で指定します。(Googleマップも同様)
・zoomを0~18で指定(Googleマップは「0~21」)タイルによって違う
・経緯度の指定方法は左手系経緯度で「緯度,経度」の順で指定します。(Googleマップも同様)
・zoomを0~18で指定(Googleマップは「0~21」)タイルによって違う
var mpoint = [35.6812362,139.7671248]; map.setView(mpoint, 16);
地図タイルの指定
・OpenStreetMapの地図タイルを指定する場合。
・OpenStreetMapの地図タイルを指定する場合。
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', { attribution: '© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors' }).addTo(map);
・国土地理院の地図タイルを指定する場合。
L.tileLayer('https://cyberjapandata.gsi.go.jp/xyz/std/{z}/{x}/{y}.png', { attribution: "<a href='https://maps.gsi.go.jp/development/ichiran.html' target='_blank'>国土地理院</a>" }).addTo(map);
・国土地理院の衛星写真タイルを指定する場合。
L.tileLayer('https://cyberjapandata.gsi.go.jp/xyz/seamlessphoto/{z}/{x}/{y}.jpg', { attribution: "<a href='https://maps.gsi.go.jp/development/ichiran.html' target='_blank'>国土地理院</a>" }).addTo(map);
マーカーをセット
・mapのmpoint位置にマーカーをセット
・mapのmpoint位置にマーカーをセット
var marker = L.marker(mpoint).addTo(map);
HTMLのdivタグ(地図の入れ物)を作成
・HTMLで「div id=”map1“」idをセットして、CSSのheightは必ず設定します。
・HTMLで「div id=”map1“」idをセットして、CSSのheightは必ず設定します。
<div id="map1" style="height:600px;width:95%"></div>
地図のレイヤー切り替え表示
leaflet2.html
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Leflet1</title> <link rel="stylesheet" href="https://unpkg.com/leaflet@1.7.1/dist/leaflet.css"/> <script src="https://unpkg.com/leaflet@1.7.1/dist/leaflet.js"></script> <script> function init() { var osm_std = new L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', { attribution: '© <a href="https://osm.org/copyright" target="_blank">OpenStreetMap</a> contributors' }); var tiri_std = new L.tileLayer('https://cyberjapandata.gsi.go.jp/xyz/std/{z}/{x}/{y}.png', { attribution: "<a href='https://maps.gsi.go.jp/development/ichiran.html' target='_blank'>国土地理院</a>" }); var tiri_pic = new L.tileLayer('https://cyberjapandata.gsi.go.jp/xyz/seamlessphoto/{z}/{x}/{y}.jpg', { attribution: "<a href='https://maps.gsi.go.jp/development/ichiran.html' target='_blank'>国土地理院</a>" }); var mpoint = [35.6812362,139.7671248]; var map = L.map('map1', { center: mpoint, zoom: 16, layers: [osm_std] }); var maplayer = { 'OpenStreetMap': osm_std, '地理院地図': tiri_std, '地理院写真': tiri_pic }; L.control.layers(maplayer, null).addTo(map); var marker = L.marker(mpoint).addTo(map); marker.bindTooltip('ツールチップ',{direction:'top'}); } </script> </head> <body onload="init()"> <div id="map1" style="height:600px; width:90%; margin: 0 auto;"></div> </body> </html>
WordPress上に表示する(Cocoonの場合)
head-insert.phpに追記と「地図有」カテゴリー作成
・leaflet.cssとleaflet.jsは、headerに書き込むのですがFTPを使って「/wp-content/themes/cocoon-child/tmp-user/head-insert.php」をダウンロードして下記のように青アンダーラインを追記してからアップロード
・「if (in_category(‘地図有‘))」で指定した(地図有)カテゴリーの時だけleaflet.cssとleaflet.jsを読み込みます。
・「if (in_category(‘地図有‘))」で指定した(地図有)カテゴリーの時だけleaflet.cssとleaflet.jsを読み込みます。
<?php //ヘッダー部分(<head></head>内)にタグを挿入したいときは、このテンプレートに挿入(ヘッダーに挿入する解析タグなど) //子テーマのカスタマイズ部分を最小限に抑えたい場合に有効なテンプレートとなります。 //例:<script type="text/javascript">解析コード</script> ?> <?php if (!is_user_administrator()) : //管理者を除外してカウントする場合は以下に挿入 ?> <?php endif; ?> <?php //全ての訪問者をカウントする場合は以下に挿入 ?> <?php if (in_category('地図有')) : ?> <link rel="stylesheet" href="https://unpkg.com/leaflet@1.7.1/dist/leaflet.css" /> <script src="https://unpkg.com/leaflet@1.7.1/dist/leaflet.js"></script> <?php endif; ?>
・上の例では、「地図有」カテゴリーを指定しているのでWordPressで「地図有」カテゴリーを作って地図を表示する場合は、必ず「地図有」カテゴリーに入れてください。
・カテゴリー名は何でも良いのですが、「地図有」とか「onMap」あたりが良いかと。
WordPress記事に「div」「script」を挿入
・地図を挿入したい場所で「HTML挿入」をクリック
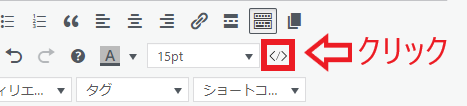
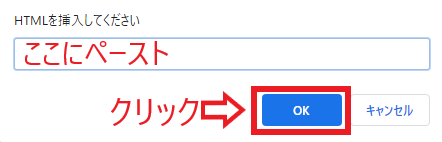
・以下をコピーペーストして「OK」をクリック(空行は無い方が良いです。)
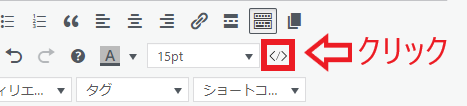
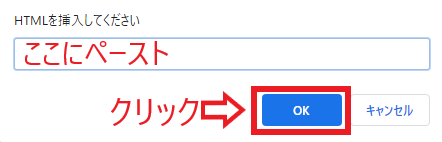
・以下をコピーペーストして「OK」をクリック(空行は無い方が良いです。)
<div id="map1" style="height: 600px; width: 90%; margin: 0 auto;"><script> function init(){ var osm_std = new L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', { attribution: '© <a href="https://osm.org/copyright" target="_blank">OpenStreetMap</a> contributors' }); var tiri_std = new L.tileLayer('https://cyberjapandata.gsi.go.jp/xyz/std/{z}/{x}/{y}.png', { attribution: "<a href='https://maps.gsi.go.jp/development/ichiran.html' target='_blank'>国土地理院</a>" }); var tiri_pic = new L.tileLayer('https://cyberjapandata.gsi.go.jp/xyz/seamlessphoto/{z}/{x}/{y}.jpg', { attribution: "<a href='https://maps.gsi.go.jp/development/ichiran.html' target='_blank'>国土地理院</a>" }); var mpoint = [35.6812362,139.7671248]; var map = L.map('map1', { center: mpoint, zoom: 16, layers: [osm_std] }); var maplayer = { 'OpenStreetMap': osm_std, '地理院地図': tiri_std, '地理院写真': tiri_pic }; L.control.layers(maplayer, null).addTo(map); var marker = L.marker(mpoint).addTo(map); marker.bindTooltip('ツールチップ',{direction:'top'}); } window.onload = function(){init();}</script></div>
・テキストビューモードで挿入しても良いのですが余計なタグが挿入される場合が有りますので注意が必要です。
参考
Leaflet — an open-source JavaScript library for interactive maps
Leaflet is a modern, lightweight open-source JavaScript library for mobile-friendly interactive maps.
地理院地図|地理院タイル一覧